Xamarin Essentials Device Info
The DeviceInfo class provides information about the device the application is running on.
Let's work with Device Info Feature provided by Xamarin Essentials
Open the base proyect (Essentials), and create a new Content Page inside Portable Proyect -> Views, with the name: DeviceInfoPage
After that just paste this code in DeviceInfoPage.xaml:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
<?xml version="1.0" encoding="utf-8" ?> <ContentPage x:Class="Essentials.Views.DeviceInfoPage" xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"> <ContentPage.Content> <Grid> <Grid.RowDefinitions> <RowDefinition Height="60" /> <RowDefinition Height="*" /> <RowDefinition Height="1" /> <RowDefinition Height="*" /> <RowDefinition Height="1" /> <RowDefinition Height="*" /> <RowDefinition Height="1" /> <RowDefinition Height="*" /> <RowDefinition Height="1" /> <RowDefinition Height="*" /> <RowDefinition Height="1" /> <RowDefinition Height="*" /> <RowDefinition Height="1" /> <RowDefinition Height="*" /> </Grid.RowDefinitions> <Label Grid.Row="0" FontSize="30" HorizontalOptions="Center" Text="Device Info" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="deviceModelLabel" Grid.Row="1" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="deviceManufacturerLabel" Grid.Row="3" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="deviceNameLabel" Grid.Row="5" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="versionLabel" Grid.Row="7" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="platformLabel" Grid.Row="9" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="idiomLabel" Grid.Row="11" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <Label x:Name="deviceTypeLabel" Grid.Row="13" FontSize="23" HorizontalOptions="Center" TextColor="{StaticResource NavigationPrimary}" VerticalOptions="Center" /> <BoxView Grid.Row="2" BackgroundColor="{StaticResource SecondColor}" /> <BoxView Grid.Row="4" BackgroundColor="{StaticResource SecondColor}" /> <BoxView Grid.Row="6" BackgroundColor="{StaticResource SecondColor}" /> <BoxView Grid.Row="8" BackgroundColor="{StaticResource SecondColor}" /> <BoxView Grid.Row="10" BackgroundColor="{StaticResource SecondColor}" /> <BoxView Grid.Row="12" BackgroundColor="{StaticResource SecondColor}" /> </Grid> </ContentPage.Content> </ContentPage> |
Now we have the View Ready, let's fill those labels with our device information !!!
Go to DeviceInfoPage.xaml.csand just paste this code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
using Xamarin.Essentials; using Xamarin.Forms; using Xamarin.Forms.Xaml; namespace Essentials.Views { [XamlCompilation(XamlCompilationOptions.Compile)] public partial class DeviceInfoPage : ContentPage { public DeviceInfoPage() { InitializeComponent(); // Device Model var device = DeviceInfo.Model; // Manufacturer var manufacturer = DeviceInfo.Manufacturer; // Device Name var deviceName = DeviceInfo.Name; // Operating System Version Number var version = DeviceInfo.VersionString; // Platform var platform = DeviceInfo.Platform; // Idiom var idiom = DeviceInfo.Idiom; // Device Type var deviceType = DeviceInfo.DeviceType; deviceModelLabel.Text = $"Device Model: {device}"; deviceManufacturerLabel.Text = $"Manufacturer: {manufacturer}"; deviceNameLabel.Text = $"Device Name: {deviceName}"; versionLabel.Text = $"Version Number: {version}"; platformLabel.Text = $"Platform: {platform}"; idiomLabel.Text = $"Idiom: {idiom}"; deviceTypeLabel.Text = $"Device Type: {deviceType}"; } } } |
That's it, the app is almost ready to play, the last step is put the page into our flyout
Above this lines of code on AppShell.xaml:
1 2 3 |
<ShellItem Title="Connectivity" FlyoutIcon="{ext:ImageResource Essentials.Resources.Images.FlyoutIcon.png}"> <ShellContent ContentTemplate="{DataTemplate local:ConnectivityPage}" /> </ShellItem> |
Add this:
1 2 3 |
<ShellItem Title="Device Info" FlyoutIcon="{ext:ImageResource Essentials.Resources.Images.FlyoutIcon.png}"> <ShellContent ContentTemplate="{DataTemplate local:DeviceInfoPage}" /> </ShellItem> |
Alright we've finished this Lab !!!
Results
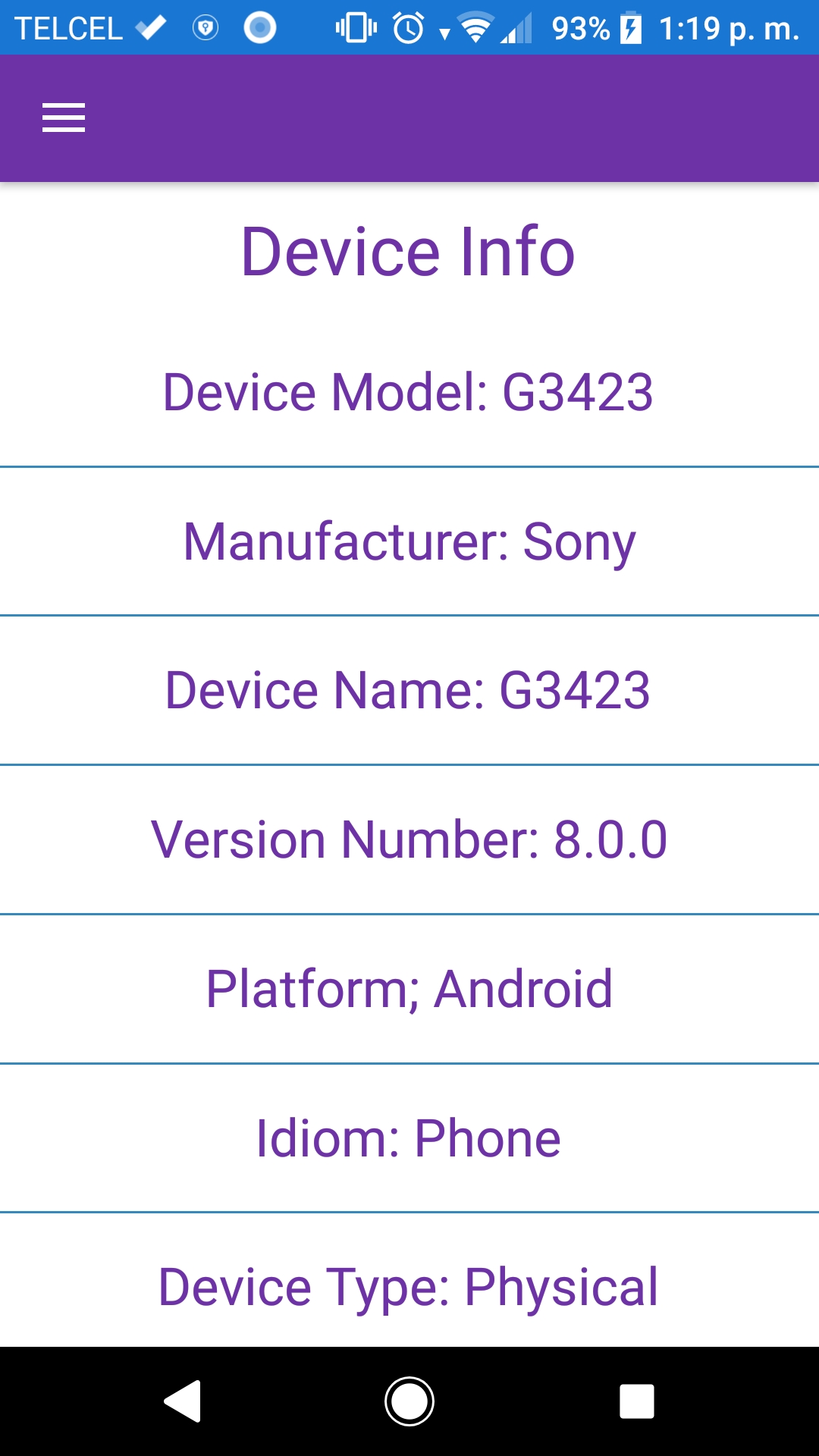
Related Links
Xamarin Essentials NuGet Essentials
Xamarin Essentials Documentation Microsoft Xamarin.Essentials Documentation
Xamarin Essentials Device Info Documentation Device Info
Completed Solution Essentials Repo