New Relic on MAUI
Introduction:
Microsoft recently announced that Visual Studio App Center will be retired on March 31, 2025. You can learn more about this announcement on Microsoft’s official roadmap page. As developers, this prompts us to explore alternative solutions for crash analytics. In this guide, I’ll walk you through setting up New Relic for crash analytics in .NET Maui apps.
What is New Relic:
According to their official page, “New Relic is an observability platform that helps you build better software. You can bring in data from any digital source so that you can fully understand your system, analyze that data efficiently, and respond to incidents before they become problems.”
Put simply, New Relic is like a toolbox for developers. It gathers information from all over your system so you can see what’s going on. It’s great for figuring out how your software is doing and spotting any issues before they cause trouble for users.
Getting Started with New Relic
Step 1: Create a New Relic Account:
Visit New Relic’s signup page to create a new account. During the signup process, choose .NET Maui under the Mobile section as the platform you want to integrate with.
Step 2: Setup Project Settings
Once you begin setting up your project, you’ll encounter a screen with steps like the one shown below. Don’t worry — I’ll guide you through each step.

- Name Your App:
When you’re setting up your project, you’ll make apps for both Android and iOS. These names are super important because they help you find your data later. Make sure to use a name that describes your project well. You can use the same name for both the Android and iOS apps, just like I did.
New Relic will add “-android” to the Android app name and “-ios” to the iOS app name to keep things organized.
For example:
- Android App Name: CrashAnalyticsPOC
- iOS App Name: CrashAnalyticsPOC
Make sure the names you choose are clear and unique so you can find your app data easily in New Relic.
- Review Requirements:
Before you install our .NET MAUI agent, it’s important to check if your app meets these version requirements:
- Your app should be using .NET version 6.0 or newer.
- If you’re building an Android app, it should be for Android 7 (API level 24) or newer.
For iOS apps:
- They should be for iOS 11 or newer.
- Make sure you’re using the latest version of Xcode.
Ensuring your app meets these criteria will ensure that it works well with the New Relic .NET MAUI agent.
- Add the .NET MAUI Agent:
To add the .NET MAUI agent to your project, follow these steps:
1. Open your .NET MAUI solution and select the project you want to add the agent to.
2. Open the context menu for the selected project.
3. Click on “Add” > “Add NuGet packages.”
4. Search for “NewRelic.MAUI.Plugin” and select it.
This will show you a popup like the one given below. Hit accept, and all these packages will be installed into your project.

Install the “NewRelic.MAUI.Plugin” NuGet package into your Maui Project for integration. It seems to be working fine for iOS and Android as of now. You can now proceed to check it.
Step 3: Configuration
- Copy Your Application Token:
Obtain a unique application token for iOS and Android. The application token is used for New Relic to authenticate your .NET MAUI app’s data. As we have already created separate apps for Android and iOS, we will find individual app tokens for each operating system here.
Copy your app token. You’ll need this token for the next step.
In the future, if you need to find your app token, you can follow these steps:
1. Go to one.newrelic.com, click Mobile in your left navigation, or scroll to your mobile apps in the All Entities list.
2. Select your .NET MAUI app.
3. Go to Settings > Application and copy the displayed Application token.
- Add Agent Configuration:
Configure the agent in your .NET Maui project to handle uncaught exceptions and track shell navigated events. Here’s the code snippet you need to add to your ‘App.cs’ file:
using NewRelic.MAUI.Plugin;
...
public App()
{
InitializeComponent();
MainPage = new AppShell();
CrossNewRelic.Current.HandleUncaughtException();
CrossNewRelic.Current.TrackShellNavigatedEvents();
// Set optional agent configuration
// Options are: crashReportingEnabled, loggingEnabled, logLevel, collectorAddress, crashCollectorAddress
AgentStartConfiguration agentConfig = new AgentStartConfiguration(true, true, LogLevel.INFO, "mobile-collector.newrelic.com", "mobile-crash.newrelic.com");
if (DeviceInfo.Current.Platform == DevicePlatform.Android)
{
// Start with agent configuration
CrossNewRelic.Current.Start("<YOUR_ANDROID_TOKEN>", agentConfig);
}
else if (DeviceInfo.Current.Platform == DevicePlatform.iOS)
{
// Start with agent configuration
CrossNewRelic.Current.Start("<YOUR_IOS_TOKEN>", agentConfig);
}
}
```
This code will enable New Relic to handle uncaught exceptions and track shell navigated events in your .NET Maui application. Make sure to replace `<YOUR_ANDROID_TOKEN>` and `<YOUR_IOS_TOKEN>` with your actual Android and iOS app tokens obtained from New Relic.
Step 4: Permissions (Android Only)
Add the following permissions to your Android manifest file:
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.INTERNET" />
Step 5: Build and Run Your Application
Clean your project and run your app on an emulator or device to start collecting data.
- Testing Crash Scenarios:
To simulate a crash, you can use the following code snippet:
private void OnCounterClicked(object sender, EventArgs e)
{
throw new Exception("Test crash created");
}
- Logging Handled Exceptions:
To log handled exceptions in New Relic, utilize the code snippet provided below:
private void LogExceptionToNewRelic()
{
try
{
throw new Exception("Sample exception to demonstrate logging.");
}
catch (Exception ex)
{
CrossNewRelic.Current.RecordException(ex);
}
}
This code captures and logs exceptions within your application to New Relic, facilitating comprehensive error monitoring and analysis.
Screenshots for iOS:
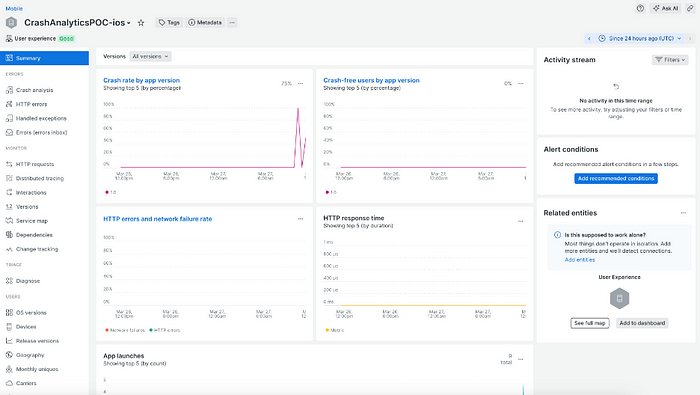


Screenshots for Android:

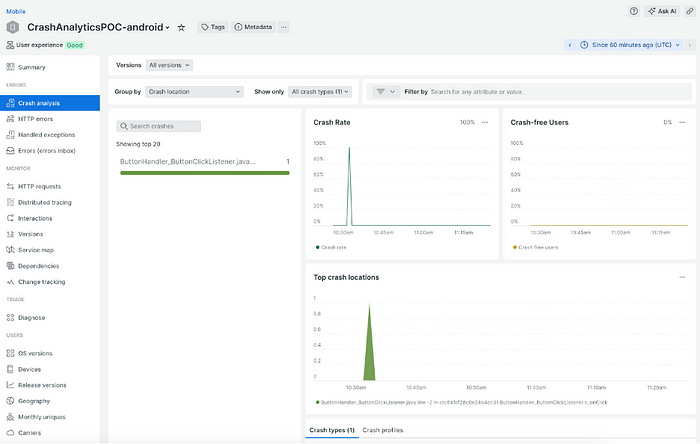

Conclusion
Integrating New Relic for crash analytics in .NET Maui applications provides developers with a powerful tool to monitor and analyze app performance effectively. By following the steps outlined in this guide, you can seamlessly integrate New Relic into your projects, ensuring better software quality and user experience.